Do want to make some HTTP request to some enpoint but not able to make because of Browser feature, which is called CORS.
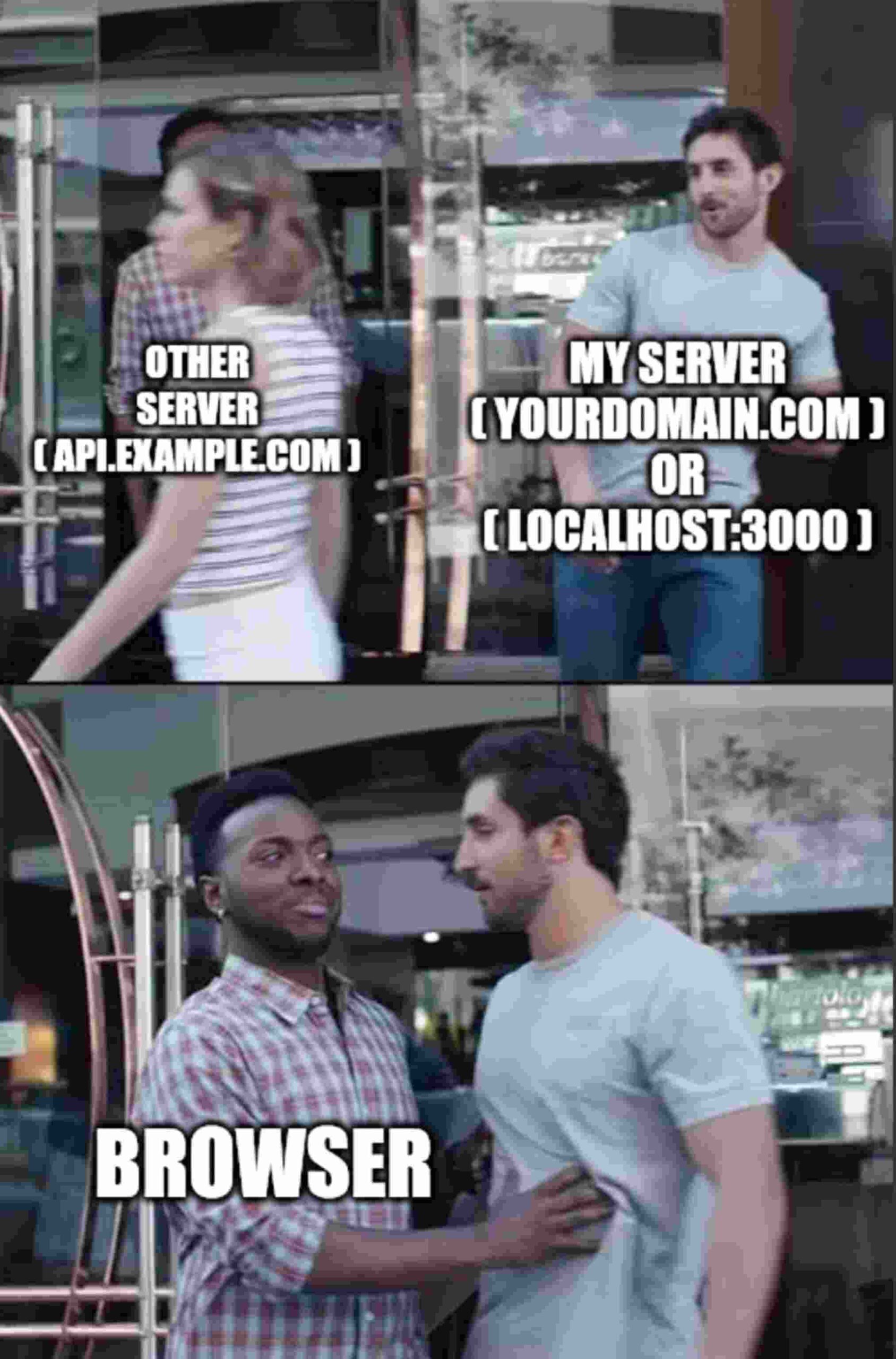
CORS, or Cross-Origin Resource Sharing, is a security feature implemented by web browsers to control how resources on a web page can be requested from another domain outside the domain from which the resource originated.
Example:
My domain is localhost:3000 and Other domain is api.example.com . So now if i want to make a request from my local server to other server which have different domain. Then a flow of CORS request will started.
Flow of CORS request :
1. Initial Request:
You (the client) attempt to make a request to a resource (like an API endpoint) located on a different domain (let’s call it https://api.example.com) from the domain of your current page eg.( localhost:3000 ).
2. Preflight Request:
Condition: If your request is considered a “complex request” by the browser, meaning it uses methods other than GET, HEAD, or POST, or it includes headers other than simple headers (like Content-Type), the browser will automatically initiate a preflight request.
Preflight Request: The browser sends an HTTP OPTIONS request to the server (https://api.example.com) to determine if the actual request (e.g., POST, PUT, DELETE) is safe to send. This preflight request includes headers such as Origin, Access-Control-Request-Method, and Access-Control-Request-Headers.
– Server Response: The server (https://api.example.com) must respond to this preflight request with appropriate CORS headers:
– Access-Control-Allow-Origin: Specifies which origins are allowed to access the resource (* for all, or specific domains like https://www.yourwebsite.com).
– Access-Control-Allow-Methods: Lists the allowed HTTP methods for accessing the resource (GET, POST, PUT, etc.).
– Access-Control-Allow-Headers: Lists the allowed headers that can be used in the actual request.
The preflight request ensures that potential cross-origin requests are safe and authorized before the actual data exchange occurs, maintaining security and integrity on the web.
3. Actual Request (if approved):
Condition: If the server responds to the preflight request with appropriate CORS headers and indicates that the actual request is allowed (Access-Control-Allow-Origin includes the origin of your request and any required methods/headers are allowed), then the browser proceeds to send the actual request (e.g., POST, PUT, DELETE) to https://api.example.com.
Which means that https://api.example.com server is allowing you to make that request and you can access that.
– Server Response: The server (https://api.example.com) processes the request and sends back the response.
4. Handling Responses:
Success: If the server responds with a successful status (e.g., 200 OK), the browser allows your client application to access the response data.
Error: If there are CORS-related errors (e.g., mismatch in allowed origin, methods, or headers), the browser blocks the response data from being accessed by your client application, adhering to the same-origin policy.
Solution of CORS error :
So because of browser built-in security feature we are not able to make a request on different domain. But we can bypass this using plugin and some extensions but that not right approach, it can break the security of browsers and sometimes these things not work well.
Now for developer community i made something interesting which is called cors-handler. It basically act as a proxy sever and will make request on give url path or domain which you want to access. It will not give you any kind of CORS related errors because in our server setting configuration we are allowing all the origins to access our services.
Now you don’t have to make direct HTTP request to another domain or path-url to access something, use our path-url to access another domain :
“https://handler-cors.vercel.app/fetch“
Note: I’m helping the developer community and no any charges will be taken from you. It is a free service and I’ll maintain it for you guys.
How to use cors-handler??
Make sure that you use right endpoint which is /fetch, make POST request and also send a url key field in body object with its value.
We have provided some example using fetch and axios in these examples you just have to change the “https://www.example.com/api/endpoint” with right url which you want to access.
You can use any approach from given examples:
– Using fetch
//Define an async function to make the POST request async function getData() { try { const res = await fetch("https://handler-cors.vercel.app/fetch", { method: "POST", headers: { "Content-Type": "application/json", }, body: JSON.stringify({ url: "https://www.example.com/api/endpoint", //Replace this example url with right url which you want to access }) }); if (!res.ok) { throw new Error(`Error: ${res.status} ${res.statusText}`); } const raw = await res.json(); console.log(raw); // see raw in console } catch (error) { console.error("Error fetching data:", error); } } getData(); //calling the function
– Using axios
const axios = require('axios'); // install axios or use can use CDN Link of it // Define the data to be sent const bodyData = { url: 'https://example.com/api/endpoint', //Replace this example url with right url which you want }; // Define the config with headers const config = { headers: { 'Content-Type': 'application/json', } }; // Define an async function to make the POST request async function getData() { try { const response = await axios.post("https://handler-cors.vercel.app/fetch", bodyData, config); console.log('Response:', response.data); } catch (error) { console.error('Error:', error); } } getData() // calling the function
1,123 Comments
I’d need to test with you here. Which isn’t one thing I usually do! I take pleasure in studying a submit that can make individuals think. Additionally, thanks for permitting me to remark!
Thanks again for the article.Really looking forward to read more. Keep writing.
pretty useful material, overall I think this is well worth a bookmark, thanks
Many thanks for sharing your feelings on meta_keyword. Regards
Thanks , I’ve just been looking for info approximately this topic for a longtime and yours is the greatest I’ve found out so far.But, what about the conclusion? Are you positive concerning the supply?
Normally I don’t read article on blogs, but I wouldlike to say that this write-up very compelled me to take a look at and do it!Your writing style has been surprised me. Thanks,quite nice post.my blog post – click to find out more
I loved your blog post.Really looking forward to read more. Really Great.
Im grateful for the article.Really thank you! Cool.
Thanks for sharing, this is a fantastic blog post.Much thanks again. Really Cool.
I am so grateful for your post.Really looking forward to read more.
Wow, great blog post. Will read on…
Muchos Gracias for your blog article.Thanks Again. Really Cool.
Great, thanks for sharing this blog article.Really looking forward to read more. Really Great.
Thanks for the marvelous posting! I definitely enjoyed reading it, you might be a great author.I will make sure to bookmark your blog and will often come back later on. I want to encourage continue your great writing, have a nice day!
What’s Happening i’m new to this, I stumbled upon this I have discovered It positively useful and it has helped me out loads. I’m hoping to give a contribution & aid other customers like its helped me. Good job.
monster resume writing service reviewtop resume writing companies
Very nice post and right to the point. I am not sure if this is actually the best place to ask but do you people have any thoughts on where to employ some professional writers? Thx 🙂
chloroquine phosphate tablet chloroquine phosphate over the counter
If some one wants expert view about running a blog then irecommend him/her to pay a quick visit this blog, Keep up the nice job.
Enjoyed looking through this, very good stuff, regards . “Management is nothing more than motivating other people.” by Lee Iacocca.
ivermectin for sale near me ivermectin autoimmune
acquistare farmaci senza ricetta: Farma Prodotti – farmacie online sicure
acquisto farmaci con ricetta
This is one awesome blog post.Really looking forward to read more. Awesome.
Can you be more specific about the content of your article? After reading it, I still have some doubts. Hope you can help me.
Heya! I’m at work browsing your blog from my new iphone 4!Just wanted to say I love reading your blog and look forward to all your posts!Carry on the fantastic work!
Hi! I just wish to give you a big thumbs up for the excellent information you have got right here on this post. I’ll be coming back to your blog for more soon.
Farmacia online miglior prezzo: Cialis senza ricetta – acquistare farmaci senza ricetta
phtaya phtaya.tech Casino promotions draw in new players frequently.
https://phmacao.life/# Many casinos host charity events and fundraisers.
Entertainment shows are common in casinos.
Many casinos host charity events and fundraisers.: taya777 login – taya777 login
Live music events often accompany gaming nights.: taya777 app – taya777 register login
taya777 taya777 login High rollers receive exclusive treatment and bonuses.
The casino experience is memorable and unique.: taya365 login – taya365 com login
winchile casino winchile La pasiГіn por el juego une a personas.
http://jugabet.xyz/# La Г©tica del juego es esencial.
The Philippines offers a rich gaming culture.
http://winchile.pro/# Las promociones de fin de semana son populares.
Gaming regulations are overseen by PAGCOR.
Las experiencias son Гєnicas en cada visita.: jugabet – jugabet.xyz
https://taya365.art/# Loyalty programs reward regular customers generously.
The Philippines has several world-class integrated resorts.
winchile casino winchile.pro Los casinos celebran festivales de juego anualmente.
https://jugabet.xyz/# Los casinos organizan eventos especiales regularmente.
Players must be at least 21 years old.
http://taya777.icu/# Loyalty programs reward regular customers generously.
Many casinos offer luxurious amenities and services.
Los jugadores pueden disfrutar desde casa.: winchile casino – win chile
taya777 app taya777 Most casinos offer convenient transportation options.
https://taya365.art/# Many casinos have beautiful ocean views.
Casino promotions draw in new players frequently.
La pasiГіn por el juego une a personas.: jugabet – jugabet.xyz
http://phtaya.tech/# Responsible gaming initiatives are promoted actively.
Casino promotions draw in new players frequently.
jugabet casino jugabet casino Las mГЎquinas tragamonedas tienen temГЎticas diversas.
https://taya777.icu/# Many casinos offer luxurious amenities and services.
The casino scene is constantly evolving.
http://jugabet.xyz/# Muchos casinos ofrecen restaurantes y bares.
Players often share tips and strategies.
http://phtaya.tech/# Some casinos have luxurious spa facilities.
Loyalty programs reward regular customers generously.
Gambling regulations are strictly enforced in casinos.: phmacao – phmacao com login
http://winchile.pro/# Algunos casinos tienen programas de recompensas.
Slot tournaments create friendly competitions among players.
La iluminaciГіn crea un ambiente vibrante.: jugabet casino – jugabet.xyz
https://winchile.pro/# La mГєsica acompaГ±a la experiencia de juego.
Loyalty programs reward regular customers generously.
win chile winchile La mГєsica acompaГ±a la experiencia de juego.
http://taya777.icu/# Players must be at least 21 years old.
Poker rooms host exciting tournaments regularly.
Many casinos offer luxurious amenities and services.: phtaya casino – phtaya casino
phmacao club phmacao.life The Philippines has several world-class integrated resorts.
http://phtaya.tech/# Players must be at least 21 years old.
The casino experience is memorable and unique.
Casinos often host special holiday promotions.: phmacao – phmacao casino
http://taya777.icu/# Slot tournaments create friendly competitions among players.
Online gaming is also growing in popularity.
Many casinos offer luxurious amenities and services. http://winchile.pro/# La iluminaciГіn crea un ambiente vibrante.
Visitors come from around the world to play.: phtaya.tech – phtaya login
taya365 login taya365 com login The poker community is very active here.
https://jugabet.xyz/# Hay reglas especГficas para cada juego.
The Philippines has several world-class integrated resorts.
Los casinos celebran festivales de juego anualmente.: winchile casino – winchile.pro
http://winchile.pro/# Muchos casinos tienen salas de bingo.
Slot tournaments create friendly competitions among players.
Aw, this was an incredibly nice post. Taking a few minutes and actual effort to create a great articleÖ but what can I sayÖ I put things off a whole lot and don’t manage to get anything done.
https://phtaya.tech/# The casino industry supports local economies significantly.
Gaming regulations are overseen by PAGCOR.
Los casinos son lugares de reuniГіn social.: jugabet chile – jugabet.xyz
win chile winchile Los juegos en vivo ofrecen emociГіn adicional.
Las ganancias son una gran motivaciГіn.: winchile – winchile.pro
http://phmacao.life/# Manila is home to many large casinos.
Players can enjoy high-stakes betting options.
La adrenalina es parte del juego.: jugabet – jugabet chile
http://taya777.icu/# Casino visits are a popular tourist attraction.
High rollers receive exclusive treatment and bonuses.
family pharmacy family pharmacy Best online pharmacy
cheapest pharmacy for prescription drugs https://megaindiapharm.shop/# Mega India Pharm
mexican border pharmacies shipping to usa: xxl mexican pharm – xxl mexican pharm
discount drug mart: drug mart – canadian pharmacy coupon code
cheapest prescription pharmacy https://megaindiapharm.com/# MegaIndiaPharm
canadian pharmacy scam easy canadian pharm easy canadian pharm
mail order pharmacy no prescription https://familypharmacy.company/# Cheapest online pharmacy
canadian pharmacy discount coupon: online pharmacy delivery usa – Online pharmacy USA
canadian pharmacy no prescription http://familypharmacy.company/# Cheapest online pharmacy
discount drug mart discount drug mart pharmacy drug mart
xxl mexican pharm: xxl mexican pharm – xxl mexican pharm
canada pharmacy coupon https://familypharmacy.company/# non prescription medicine pharmacy
Best online pharmacy: Best online pharmacy – Online pharmacy USA
prescription drugs online https://familypharmacy.company/# canadian pharmacy coupon code
mail order prescription drugs from canada https://megaindiapharm.com/# Mega India Pharm
canadian pharmacy without prescription: discount drug pharmacy – discount drug pharmacy
canadian pharmacy coupon https://xxlmexicanpharm.com/# mexican pharmaceuticals online
cheapest prescription pharmacy: online pharmacy delivery usa – Online pharmacy USA
Thank you, I ave recently been searching for information about this topic for ages and yours is the best I have discovered till now. But, what about the bottom line? Are you sure about the source?
pharmacy coupons https://megaindiapharm.com/# Mega India Pharm
An impressive share, I just given this onto a colleague who was doing a little analysis on this. And he in fact bought me breakfast because I found it for him.. smile. So let me reword that: Thnx for the treat! But yeah Thnkx for spending the time to discuss this, I feel strongly about it and love reading more on this topic. If possible, as you become expertise, would you mind updating your blog with more details? It is highly helpful for me. Big thumb up for this blog post!
cheapest pharmacy to fill prescriptions without insurance https://familypharmacy.company/# Online pharmacy USA
drug mart drug mart drugmart
Nicely put. Thanks a lot!essay homework help writing paper find a ghostwriter
purple pharmacy mexico price list: п»їbest mexican online pharmacies – п»їbest mexican online pharmacies
legit non prescription pharmacies https://xxlmexicanpharm.com/# xxl mexican pharm
Thank you for your sharing. I am worried that I lack creative ideas. It is your article that makes me full of hope. Thank you. But, I have a question, can you help me?
cheapest pharmacy prescription drugs https://familypharmacy.company/# Cheapest online pharmacy
mexico drug stores pharmacies: buying prescription drugs in mexico – xxl mexican pharm
I’m still learning from you, but I’m trying to achieve my goals.I certainly enjoy reading everything that is written on your blog.Keep the aarticles coming.I loved it!
xxl mexican pharm: mexican rx online – xxl mexican pharm
rx pharmacy no prescription https://megaindiapharm.shop/# indianpharmacy com
It’s really a great and helpful piece of information. I am happy that you just shared this useful info with us. Please stay us up to date like this. Thanks for sharing.
Mega India Pharm: MegaIndiaPharm – MegaIndiaPharm
cheapest pharmacy to get prescriptions filled https://discountdrugmart.pro/# online pharmacy non prescription drugs
international pharmacy no prescription http://easycanadianpharm.com/# my canadian pharmacy review
cheapest pharmacy to fill prescriptions without insurance https://easycanadianpharm.com/# canadianpharmacymeds com
online pharmacy delivery usa Best online pharmacy family pharmacy
prescription free canadian pharmacy https://familypharmacy.company/# Online pharmacy USA
Online pharmacy USA: Best online pharmacy – online pharmacy delivery usa
best no prescription pharmacy https://megaindiapharm.com/# MegaIndiaPharm
no prescription needed pharmacy https://xxlmexicanpharm.shop/# buying prescription drugs in mexico
discount drug mart pharmacy discount drug mart pharmacy drugmart
cheap pharmacy no prescription https://familypharmacy.company/# online pharmacy delivery usa
top 10 online pharmacy in india: MegaIndiaPharm – buy medicines online in india
canadian pharmacy review: easy canadian pharm – easy canadian pharm
Hello there, You’ve done a great job. I will definitely digg it and in my opinion suggest to my friends. I’m confident they will be benefited from this site.
online canadian pharmacy coupon https://xxlmexicanpharm.com/# xxl mexican pharm
online pharmacy delivery usa online pharmacy delivery usa Best online pharmacy
cheapest pharmacy for prescriptions https://xxlmexicanpharm.com/# xxl mexican pharm
canadian pharmacy no prescription needed http://easycanadianpharm.com/# easy canadian pharm
canadian pharmacy world coupons https://megaindiapharm.com/# top 10 online pharmacy in india
discount drug pharmacy discount drugs drugmart
I like this web blog very much, Its a very nice berth to read and incur information. “God cannot alter the past, but historians can.” by Samuel Butler.
Banyak pemain menikmati jackpot harian di slot http://bonaslot.site/# Jackpot progresif menarik banyak pemain
Kasino memastikan keamanan para pemain dengan baik: preman69.tech – preman69.tech
This reminds me of the other info I was seeing earlier
Mesin slot dapat dimainkan dalam berbagai bahasa http://slotdemo.auction/# Kasino selalu memperbarui mesin slotnya
You made a few nice points there. I did a search on the issue and found mainly persons will agree with your blog.
Pemain sering berbagi tips untuk menang https://slot88.company/# Kasino sering memberikan hadiah untuk pemain setia
Kasino menyediakan layanan pelanggan yang baik: garuda888.top – garuda888 slot
Mesin slot sering diperbarui dengan game baru https://bonaslot.site/# Kasino sering memberikan hadiah untuk pemain setia
Jackpot besar bisa mengubah hidup seseorang: bonaslot – BonaSlot
You made some decent factors there. I seemed on the internet for the issue and found most people will go together with along with your website.
Mesin slot dapat dimainkan dalam berbagai bahasa: slot88 – slot 88
I do not even know the way I stopped up here, however I assumed this publish was once great. I do not know who you might be however certainly you’re going to a famous blogger in the event you aren’t already 😉 Cheers!
Mesin slot menawarkan berbagai tema menarik https://garuda888.top/# Kasino sering mengadakan turnamen slot menarik
BonaSlot BonaSlot Slot dengan grafis 3D sangat mengesankan
You actually make it seem so easy with your presentation however I to find this matter to be actually something that I think I would never understand. It sort of feels too complicated and extremely wide for me. I am taking a look forward to your next publish, I will try to get the hang of it!
You actually make it appear really easy together with your presentation however I find this matter to be actually one thing which I think I would by no means understand. It kind of feels too complicated and very extensive for me. I’m taking a look ahead in your next post, I will attempt to get the hang of it!
Slot menawarkan berbagai jenis permainan bonus: slot demo – akun demo slot
Slot dengan pembayaran tinggi selalu diminati https://slot88.company/# Kasino menawarkan pengalaman bermain yang seru
I was just searching for this information for a while. After 6 hours of continuous Googleing, finally I got it in your web site. I wonder what is the lack of Google strategy that don’t rank this kind of informative web sites in top of the list. Generally the top sites are full of garbage.
Would you be fascinated about exchanging links?
Jackpot progresif menarik banyak pemain http://bonaslot.site/# Slot dengan bonus putaran gratis sangat populer
Slot dengan grafis 3D sangat mengesankan http://bonaslot.site/# Slot dengan grafis 3D sangat mengesankan
Kasino sering memberikan hadiah untuk pemain setia: bonaslot.site – bonaslot
garuda888 slot garuda888.top Kasino sering memberikan hadiah untuk pemain setia
Banyak pemain menikmati jackpot harian di slot https://slot88.company/# Slot menjadi daya tarik utama di kasino
Kasino memastikan keamanan para pemain dengan baik: BonaSlot – bonaslot
Kasino sering mengadakan turnamen slot menarik https://slot88.company/# Kasino di Indonesia menyediakan hiburan yang beragam
Mesin slot sering diperbarui dengan game baru: bonaslot.site – bonaslot
Well I truly enjoyed reading it. This tip procured by you is very helpful for correct planning.
preman69.tech preman69 Banyak kasino memiliki program loyalitas untuk pemain
Heya! I’m at work browsing your blog from my new apple iphone! Just wanted to say I love reading your blog and look forward to all your posts! Keep up the outstanding work!
I like the valuable information you providefor your articles. I’ll bookmark your blog and checkonce more here frequently. I’m moderately sureI will be informed a lot of new stuff proper here! Best of luck for the next!
When I initially commented I clicked the “Notify me when new comments are added” checkbox and now each time a comment is added I get several emails with the same comment. Is there any way you can remove me from that service? Thanks a lot!
Banyak pemain berusaha untuk mendapatkan jackpot: slot88 – slot88
Jackpot besar bisa mengubah hidup seseorang http://preman69.tech/# Banyak pemain menikmati bermain slot secara online
It’s actually a cool and useful piece of info. I am glad that you shared this useful information with us. Please keep us up to date like this. Thanks for sharing.
Banyak kasino memiliki program loyalitas untuk pemain http://bonaslot.site/# Kasino memastikan keamanan para pemain dengan baik
http://bonaslot.site/# Banyak kasino memiliki program loyalitas untuk pemain
demo slot pg slot demo gratis Slot memberikan kesempatan untuk menang besar
Keseruan bermain slot selalu menggoda para pemain: bonaslot – bonaslot
I was suggested this blog by my cousin. I’m not sure whether this post is written by him as no one else know such detailed about my problem. You’re incredible! Thanks!
Mesin slot menawarkan berbagai tema menarik http://slot88.company/# Slot modern memiliki grafik yang mengesankan
Permainan slot bisa dimainkan dengan berbagai taruhan: preman69 – preman69.tech
Mesin slot menawarkan berbagai tema menarik http://slot88.company/# Pemain sering mencoba berbagai jenis slot
Kasino sering memberikan hadiah untuk pemain setia: demo slot pg – slot demo
garuda888 slot garuda888.top Slot dengan grafis 3D sangat mengesankan
Banyak kasino memiliki promosi untuk slot: slot 88 – slot88
Slot menawarkan berbagai jenis permainan bonus http://bonaslot.site/# Bermain slot bisa menjadi pengalaman sosial
Kasino di Bali menarik banyak pengunjung: garuda888.top – garuda888 slot
slotdemo akun demo slot Pemain harus menetapkan batas saat bermain
Slot menjadi daya tarik utama di kasino https://slot88.company/# Pemain bisa menikmati slot dari kenyamanan rumah
Bermain slot bisa menjadi pengalaman sosial https://slotdemo.auction/# Slot menawarkan berbagai jenis permainan bonus
BonaSlot BonaSlot Mesin slot baru selalu menarik minat
Banyak kasino memiliki program loyalitas untuk pemain http://slot88.company/# Banyak pemain berusaha untuk mendapatkan jackpot
Slot menjadi bagian penting dari industri kasino: preman69.tech – preman69 slot
Wow that was unusual. I just wrote an really long comment but after I clicked submit my comment didn’t appear. Grrrr… well I’m not writing all that over again. Anyway, just wanted to say wonderful blog!
Mesin slot digital semakin banyak diminati http://preman69.tech/# п»їKasino di Indonesia sangat populer di kalangan wisatawan
Banyak pemain menikmati bermain slot secara online: bonaslot – bonaslot
BonaSlot BonaSlot Pemain harus menetapkan batas saat bermain
I like the efforts you have put in this, regards for all the great articles.
zithromax online australia: zithromax prescription online – generic zithromax medicine
generic zithromax 500mg india: buy zithromax without presc – zithromax buy online no prescription
buy doxycycline 100mg online Dox Health Pharm doxycycline 100mg buy online
doxycycline 100mg cost in india: Dox Health Pharm – doxycycline 200 mg price
doxycycline 163: DoxHealthPharm – doxycycline caps 100mg
amoxicillin 500 mg price buy cheap amoxicillin online buy amoxil
cost clomid price: ClmHealthPharm – where to get clomid now
doxycycline 75 mg: Dox Health Pharm – doxycycline tablets in india
https://zithropharm.com/# zithromax 500
zithromax tablets for sale: buy zithromax online cheap – zithromax 500mg price in india
where can i get amoxicillin 500 mg: AmoHealthPharm – buy amoxicillin online with paypal
how much is amoxicillin prescription: over the counter amoxicillin canada – buy amoxicillin online no prescription
doxycycline pills price in south africa cost doxycycline tablets doxycycline over the counter india
zithromax 500mg price in india: how to buy zithromax online – buy zithromax 500mg online
cost of cheap clomid without a prescription: ClmHealthPharm – where can i get clomid without rx
zithromax for sale us: zithromax price canada – buy zithromax 500mg online
can i buy generic clomid without prescription: ClmHealthPharm – where to buy clomid pill
zithromax 1000 mg pills ZithroPharm where to get zithromax
can you buy amoxicillin over the counter in canada: AmoHealthPharm – amoxicillin no prescipion
where buy clomid without a prescription: ClmHealthPharm – get generic clomid without dr prescription
cost of amoxicillin: AmoHealthPharm – can you buy amoxicillin over the counter canada
can i buy zithromax over the counter in canada: zithromax generic price – zithromax order online uk
doxycycline 100g tablets Dox Health Pharm cheapest doxycycline without prescrtiption
zithromax 500 mg for sale: can i buy zithromax online – zithromax azithromycin
where can i buy zithromax medicine: ZithroPharm – zithromax 500 mg for sale
amoxicillin 250 mg capsule: amoxicillin 775 mg – ampicillin amoxicillin
cost of generic doxycycline: doxycycline tablet – doxycycline pharmacy uk
pharmacie en ligne avec ordonnance: Cialis sans ordonnance 24h – vente de mГ©dicament en ligne
pharmacie en ligne france livraison belgique https://tadalafilmeilleurprix.shop/# trouver un mГ©dicament en pharmacie
https://pharmaciemeilleurprix.com/# п»їpharmacie en ligne france
pharmacie en ligne livraison europe
pharmacie en ligne pas cher: Acheter Cialis – pharmacie en ligne pas cher
pharmacie en ligne fiable: kamagra gel – pharmacie en ligne france livraison internationale
https://tadalafilmeilleurprix.shop/# pharmacie en ligne france fiable
acheter mГ©dicament en ligne sans ordonnance
vente de mГ©dicament en ligne: pharmacie en ligne france – Pharmacie en ligne livraison Europe
https://pharmaciemeilleurprix.shop/# acheter mГ©dicament en ligne sans ordonnance
pharmacie en ligne avec ordonnance
Pharmacie Internationale en ligne Acheter Cialis Achat mГ©dicament en ligne fiable
Viagra Pfizer sans ordonnance: Viagra pharmacie – Viagra homme prix en pharmacie sans ordonnance
trouver un mГ©dicament en pharmacie: pharmacie en ligne livraison europe – acheter mГ©dicament en ligne sans ordonnance
Pharmacie en ligne livraison Europe Tadalafil sans ordonnance en ligne acheter mГ©dicament en ligne sans ordonnance
pharmacie en ligne sans ordonnance: achat kamagra – vente de mГ©dicament en ligne
pharmacie en ligne avec ordonnance: cialis generique – pharmacie en ligne livraison europe
Viagra pas cher inde: Viagra pharmacie – Le gГ©nГ©rique de Viagra
п»їpharmacie en ligne france: pharmacie en ligne – п»їpharmacie en ligne france
That is a great tip especially to those new to the blogosphere.Brief but very accurate info… Thank you for sharing this one.A must read post!
pharmacie en ligne france livraison internationale: pharmacie en ligne france – pharmacie en ligne pas cher
https://kamagrameilleurprix.com/# pharmacies en ligne certifiГ©es
pharmacie en ligne avec ordonnance
Say, you got a nice article post.Really thank you! Cool.
pharmacie en ligne france fiable: trouver un mГ©dicament en pharmacie – Pharmacie Internationale en ligne
pharmacie en ligne france pas cher pharmacie en ligne vente de mГ©dicament en ligne
https://pharmaciemeilleurprix.com/# pharmacie en ligne
pharmacie en ligne france fiable
Pharmacie en ligne livraison Europe: kamagra en ligne – acheter mГ©dicament en ligne sans ordonnance
This is a great tip especially to those new to the blogosphere. Brief but very accurate info… Appreciate your sharing this one. A must read post!
pharmacie en ligne livraison europe: acheter kamagra site fiable – pharmacie en ligne
http://pharmaciemeilleurprix.com/# Pharmacie en ligne livraison Europe
pharmacie en ligne
pharmacie en ligne pas cher https://kamagrameilleurprix.shop/# pharmacie en ligne pas cher
Prix du Viagra 100mg en France: Viagra en france livraison rapide – Viagra homme sans prescription
http://viagrameilleurprix.com/# Viagra gГ©nГ©rique sans ordonnance en pharmacie
trouver un mГ©dicament en pharmacie
Pharmacie sans ordonnance: Cialis sans ordonnance 24h – pharmacie en ligne france fiable
land o lakes apartments sunscape apartments arvada apartments
pharmacies en ligne certifiГ©es: Achat mГ©dicament en ligne fiable – Pharmacie sans ordonnance
pharmacie en ligne fiable Acheter Cialis pharmacie en ligne livraison europe
pharmacie en ligne france livraison internationale https://kamagrameilleurprix.shop/# pharmacie en ligne fiable
п»їpharmacie en ligne france: kamagra oral jelly – pharmacie en ligne france fiable
pharmacie en ligne france livraison belgique: pharmacie en ligne france – pharmacie en ligne avec ordonnance
vente de mГ©dicament en ligne achat kamagra pharmacie en ligne france livraison internationale
Pharmacie Internationale en ligne: Tadalafil sans ordonnance en ligne – pharmacie en ligne france fiable
pharmacie en ligne france fiable: kamagra oral jelly – pharmacie en ligne france pas cher
https://pharmaciemeilleurprix.shop/# vente de mГ©dicament en ligne
Pharmacie Internationale en ligne
pharmacie en ligne france fiable: pharmacie en ligne – pharmacie en ligne
Great write-up, I¡¦m normal visitor of one¡¦s blog, maintain up the nice operate, and It is going to be a regular visitor for a long time.
That is the precise weblog for anybody who desires to seek out out about this topic. You understand a lot its nearly arduous to argue with you (not that I truly would want…HaHa). You undoubtedly put a new spin on a subject thats been written about for years. Nice stuff, simply nice!
pharmacie en ligne fiable: trouver un mГ©dicament en pharmacie – pharmacie en ligne france livraison internationale
https://pharmaciemeilleurprix.shop/# Pharmacie sans ordonnance
pharmacie en ligne livraison europe
Great, thanks for sharing this post. Really Cool.
pharmacie en ligne fiable: pharmacie en ligne avec ordonnance – Pharmacie en ligne livraison Europe
SildГ©nafil Teva 100 mg acheter: viagra en ligne – Viagra sans ordonnance pharmacie France
Viagra pas cher inde: Acheter Viagra Cialis sans ordonnance – SildГ©nafil 100 mg prix en pharmacie en France
pharmacie en ligne france livraison internationale http://kamagrameilleurprix.com/# Pharmacie Internationale en ligne
https://kamagrameilleurprix.com/# pharmacies en ligne certifiГ©es
pharmacie en ligne livraison europe
pharmacie en ligne france pas cher Pharmacies en ligne certifiees pharmacie en ligne france pas cher
https://viagrameilleurprix.com/# Viagra homme prix en pharmacie sans ordonnance
Pharmacie en ligne livraison Europe
pharmacie en ligne france fiable: pharmacie en ligne france – trouver un mГ©dicament en pharmacie
pharmacies en ligne certifiГ©es: pharmacie en ligne – pharmacie en ligne sans ordonnance
I loved your post.Really looking forward to read more.
http://tadalafilmeilleurprix.com/# pharmacie en ligne france livraison belgique
acheter mГ©dicament en ligne sans ordonnance
trouver un mГ©dicament en pharmacie: Cialis sans ordonnance 24h – pharmacies en ligne certifiГ©es
pharmacie en ligne livraison europe cialis sans ordonnance pharmacie en ligne livraison europe
https://pharmaciemeilleurprix.shop/# trouver un mГ©dicament en pharmacie
pharmacie en ligne livraison europe
Pharmacie en ligne livraison Europe: Cialis sans ordonnance 24h – pharmacie en ligne france fiable
Viagra sans ordonnance 24h suisse Viagra sans ordonnance livraison 24h Viagra homme prix en pharmacie sans ordonnance
pharmacie en ligne livraison europe: Acheter Cialis – pharmacies en ligne certifiГ©es
Viagra sans ordonnance livraison 24h: Viagra pharmacie – Viagra pas cher livraison rapide france
http://kamagrameilleurprix.com/# acheter mГ©dicament en ligne sans ordonnance
pharmacie en ligne france pas cher
He continually kept preaching about this. I will forward this information to him. Fairly certain he will havge a great read.
Pharmacie en ligne livraison Europe: achat kamagra – pharmacie en ligne france livraison internationale
great points altogether, you simply received a logo new reader. What would you recommend about your put up that you just made some days ago? Any certain?
Very informative blog article.Thanks Again. Will read on…
Hello.This article was extremely remarkable, especially because I was investigating for thoughts on this matter last Saturday.
Viagra gГ©nГ©rique sans ordonnance en pharmacie: viagra en ligne – Viagra vente libre pays
https://tadalafilmeilleurprix.shop/# pharmacie en ligne fiable
pharmacie en ligne
pharmacie en ligne pas cher: pharmacie en ligne france – pharmacie en ligne avec ordonnance
Achat mГ©dicament en ligne fiable: kamagra en ligne – pharmacie en ligne fiable
https://tadalafilmeilleurprix.shop/# pharmacies en ligne certifiГ©es
п»їpharmacie en ligne france
Viagra sans ordonnance 24h suisse: viagra sans ordonnance – SildГ©nafil 100mg pharmacie en ligne
Viagra pas cher livraison rapide france: viagra en ligne – Viagra vente libre allemagne
Pharmacie en ligne livraison Europe https://kamagrameilleurprix.shop/# Achat mГ©dicament en ligne fiable
http://pharmaciemeilleurprix.com/# vente de mГ©dicament en ligne
п»їpharmacie en ligne france
pharmacie en ligne avec ordonnance https://tadalafilmeilleurprix.com/# pharmacie en ligne pas cher
Enjoyed every bit of your article.Really thank you! Fantastic.
pharmacie en ligne livraison europe: acheter kamagra site fiable – п»їpharmacie en ligne france
https://kamagrameilleurprix.com/# pharmacie en ligne pas cher
п»їpharmacie en ligne france
pharmacie en ligne fiable http://viagrameilleurprix.com/# SildГ©nafil 100 mg prix en pharmacie en France
Viagra pas cher paris Viagra vente libre pays Viagra gГ©nГ©rique pas cher livraison rapide
pharmacie en ligne france pas cher: kamagra en ligne – vente de mГ©dicament en ligne
http://viagrameilleurprix.com/# Viagra sans ordonnance livraison 48h
pharmacie en ligne france livraison belgique
I cannot thank you enough for the article post.Thanks Again. Fantastic.
Prix du Viagra en pharmacie en France viagra en ligne Viagra pas cher inde
pharmacies en ligne certifiГ©es https://tadalafilmeilleurprix.shop/# acheter mГ©dicament en ligne sans ordonnance
http://pharmaciemeilleurprix.com/# trouver un mГ©dicament en pharmacie
Pharmacie sans ordonnance
pharmacie en ligne france livraison internationale achat kamagra pharmacie en ligne france livraison internationale
Heya i’m for the first time here. I found this board and I in finding It truly helpful & it helped me out a lot.I am hoping to present one thing again and help others likeyou helped me.
Thanks so much for the article post. Awesome.
plinko: plinko fr – plinko
Plinko Deutsch plinko plinko erfahrung
Wow, great blog article.Really thank you! Really Great.
http://plinkocasi.com/# Plinko app
pinco slot: pinco legal – pinco legal
http://pinco.legal/# pinco legal
Plinko online: Plinko casino game – Plinko game
https://pinco.legal/# pinco
plinko game plinko ball plinko germany
pinco slot: pinco – pinco slot
http://pinco.legal/# pinco legal
plinko fr: avis plinko – plinko
plinko fr: plinko – plinko ball
avis plinko: plinko game – PlinkoFr
plinko: plinko betrouwbaar – plinko nederland
http://pinco.legal/# pinco casino
plinko fr: plinko ball – plinko france
pinco slot pinco slot pinco legal
https://plinkocasinonl.shop/# plinko spelen
Best custom essay custom essay writing reviews essay writers needed
pinco.legal: pinco slot – pinco legal
plinko wahrscheinlichkeit plinko geld verdienen plinko game
Really appreciate you sharing this blog.Really thank you! Keep writing.
Plinko Deutsch: plinko geld verdienen – plinko wahrscheinlichkeit
Plinko app: Plinko online game – Plinko app
http://pinco.legal/# pinco
Plinko game for real money: Plinko casino game – Plinko app
Plinko games: Plinko games – Plinko online
https://plinkodeutsch.com/# plinko
Plinko casino game: Plinko online – Plinko-game
https://plinkocasinonl.shop/# plinko nederland
http://plinkocasinonl.com/# plinko
pinco casino pinco.legal pinco
http://plinkocasinonl.com/# plinko casino
pinco: pinco legal – pinco slot
https://plinkocasi.com/# Plinko game for real money
Plinko app: Plinko – Plinko online game
Plinko-game: Plinko game for real money – Plinko online
https://pinco.legal/# pinco legal
PlinkoFr: plinko fr – PlinkoFr
https://plinkodeutsch.com/# plinko ball
plinko wahrscheinlichkeit: plinko geld verdienen – plinko wahrscheinlichkeit
Thanks for sharing, this is a fantastic article.Thanks Again. Much obliged.
https://plinkocasi.com/# Plinko app
plinko germany plinko plinko germany
http://plinkodeutsch.com/# plinko germany
pinco: pinco – pinco casino
plinko casino: plinko ball – plinko casino
Say, you got a nice blog post.Thanks Again. Awesome.
pinco legal: pinco – pinco casino
Plinko Deutsch: plinko – plinko casino
https://pinco.legal/# pinco slot
plinko casino nederland: plinko casino – plinko
plinko nederland: plinko nl – plinko spelen
Hey there! I know this is kind of off topic but I was wondering if you knew where I could get a captcha plugin for my comment form?I’m using the same blog platform as yours and I’m having trouble finding one?Thanks a lot!
https://certpharm.com/# Legit online Mexican pharmacy
mexican pharmacy: mexico drug stores pharmacies – Cert Pharm
mexican drugstore online mexican pharmacy online Cert Pharm
https://certpharm.com/# mexican pharmacy
mexican mail order pharmacies: Mexican Cert Pharm – mexican pharmacy
https://certpharm.com/# mexican pharmacy
Cert Pharm: mexico drug stores pharmacies – Cert Pharm
Cert Pharm mexican pharmacy Cert Pharm
http://certpharm.com/# Legit online Mexican pharmacy
Best Mexican pharmacy online: mexican rx online – Legit online Mexican pharmacy
mexican pharmacy Legit online Mexican pharmacy mexican pharmaceuticals online
https://certpharm.shop/# Cert Pharm
mexican pharmacy online: Mexican Cert Pharm – medicine in mexico pharmacies
https://certpharm.com/# Mexican Cert Pharm
mexican pharmacy: mexican pharmacy online – Best Mexican pharmacy online
buying prescription drugs in mexico Cert Pharm Best Mexican pharmacy online
mexican pharmacy: mexican pharmaceuticals online – mexican pharmacy online
http://certpharm.com/# Mexican Cert Pharm
Mexican Cert Pharm: mexican pharmacy online – Legit online Mexican pharmacy
https://certpharm.com/# mexican pharmacy online
Best Mexican pharmacy online: Mexican Cert Pharm – Legit online Mexican pharmacy
Express Canada Pharm: canadian pharmacy service – legitimate canadian online pharmacies
canadian pharmacy no scripts: canadapharmacyonline com – Express Canada Pharm
ed meds online canada: canadian pharmacy mall – Express Canada Pharm
Express Canada Pharm vipps approved canadian online pharmacy Express Canada Pharm
canadian pharmacy victoza: Express Canada Pharm – canada drug pharmacy
canadian pharmacy in canada Express Canada Pharm buying from canadian pharmacies
canadian world pharmacy: escrow pharmacy canada – canadian pharmacy king
Express Canada Pharm: northwest pharmacy canada – buying from canadian pharmacies
Express Canada Pharm: canadian pharmacy prices – best canadian online pharmacy
certified canadian pharmacy Express Canada Pharm Express Canada Pharm
Express Canada Pharm: Express Canada Pharm – reputable canadian online pharmacy
Thanks so much for the article.Really thank you! Awesome.
Express Canada Pharm Express Canada Pharm online canadian pharmacy reviews
I really like and appreciate your blog post.Really looking forward to read more. Awesome.
Everything information about medication.
lisinopril tablet 10mg
They source globally to provide the best care locally.
Love their spacious and well-lit premises.
https://lisinoprilpharm24.top/
Always attuned to global health needs.
Always providing clarity and peace of mind.
order cheap cytotec without insurance
Their global pharmacists’ network is commendable.
Always delivering international quality.
where can i buy generic lisinopril prices
They have an impressive roster of international certifications.
They have a great range of holistic health products.
cost of generic clomid pill
A global name with a reputation for excellence.
Making global healthcare accessible and affordable.
lisinopril 3
Everything what you want to know about pills.
does propecia cause high cholesterol — propecia blind date propecia prix
Hi my friend! I wish to say that this article is amazing, nice written and include almost all important infos.I’d like to peer more posts like this .
Offering a global touch with every service.
can you buy clomid without prescription
They handle all the insurance paperwork seamlessly.
Impressed with their wide range of international medications.
where buy cheap cipro tablets
Every visit reaffirms why I choose this pharmacy.
They have a great range of holistic health products.
where buy generic clomid for sale
Their home delivery service is top-notch.
Always greeted with warmth and professionalism.
buy cytotec no prescription
Get here.
Their worldwide pharmacists’ consultations are invaluable.
fluoxetine tablet
Always ahead of the curve with global healthcare trends.
I’m impressed with their commitment to customer care.
can you buy cipro without insurance
The ambiance of the pharmacy is calming and pleasant.
Consistent excellence across continents.
https://lisinoprilpharm24.top/
The free blood pressure check is a nice touch.
A true asset to our neighborhood.
can i buy generic lisinopril without rx
A global name with a reputation for excellence.
Hassle-free prescription transfers every time.
buy generic cipro online
Always ahead of the curve with global healthcare trends.
I always find great deals in their monthly promotions.
no prescription arimidex online purchase
They take the hassle out of international prescription transfers.
Learn about the side effects, dosages, and interactions.
where to buy cytotec pills
Speedy service with a smile!
They have a fantastic range of supplements.
lisinopril 240
Consistency, quality, and care on an international level.
They offer the best prices on international brands.
how to get generic clomid
Quick service without compromising on quality.
Fast From India Fast From India Fast From India
top 10 pharmacies in india
does ivermectin kill ringworm in cattle ivermectin for ducks
Fast From India: indian pharmacy – Fast From India
It’s exhausting to seek out knowledgeable folks on this matter, but you sound like you already know what you’re speaking about! Thanks
Fast From India reputable indian online pharmacy india online pharmacy
reputable indian pharmacies
Fast From India: indianpharmacy com – Fast From India
Fast From India: Fast From India – Fast From India
top online pharmacy india: buy medicines online in india – Fast From India
Fast From India Fast From India pharmacy website india
world pharmacy india
can i drink a beer while on azithromycin – azithromycin walgreens over the counter zithromax and breastfeeding
pharmacie en ligne avec ordonnance: pharmacie en ligne avec ordonnance – pharmacie en ligne
vente de mГ©dicament en ligne Pharma Internationale Pharma Internationale
acheter mГ©dicament en ligne sans ordonnance: pharmacie en ligne pas cher – Pharma Internationale
Livescore.gs is a well-known livescore service, with quickly and on the net scores shown to any device.
Pharma Internationale: Pharmacie Internationale en ligne – Pharma Internationale
Pharma Internationale: Achat mГ©dicament en ligne fiable – Pharma Internationale
Pharma Internationale pharmacie en ligne sans ordonnance Pharma Internationale
Farmacia Medic Farmacia Medic Farmacia Medic
farmacia online barcelona: farmacias online seguras en espaГ±a – Farmacia Medic
farmacia online espaГ±a envГo internacional Farmacia Medic Farmacia Medic
You expressed that effectively.how to write essay for college application jiskha homework help write my essay service
Farmacia Medic: Farmacia Medic – Farmacia Medic
Very good post. Will read on…
Awesome blog article.Really looking forward to read more. Really Great.!!!!Loading…
Top Max Farma Farmacie online sicure Top Max Farma
scoliosisI needed to thank you for this very good read!!I certainly loved every bit of it. I have gotyou book-marked to look at new things you post… scoliosis
Top Max Farma: top farmacia online – acquisto farmaci con ricetta
acquistare farmaci senza ricetta Top Max Farma Top Max Farma
Farmacie online sicure: Top Max Farma – farmacie online autorizzate elenco
Your way of describing everything in this post is genuinely pleasant, every one be capable of without difficultyunderstand it, Thanks a lot.
Top Max Farma: Top Max Farma – farmacie online autorizzate elenco
https://canadianpharmacyaapd.com/# canadian world pharmacy
indian pharmacy
https://canadianpharmacyaapd.com/# thecanadianpharmacy
reputable indian online pharmacy
vipps canadian pharmacy canadian pharmacy meds review canadian pharmacy no scripts
http://mexicanpharmacyacp.com/# mexican drugstore online
indian pharmacy paypal
indian pharmacy Indian Pharmacy Abp India pharmacy ship to USA
Online medicine home delivery: indian pharmacy – IndianPharmacyAbp
Indian pharmacy international shipping IndianPharmacyAbp Indian pharmacy international shipping
indian pharmacy: India pharmacy ship to USA – Indian pharmacy online
https://indianpharmacyabp.com/# India pharmacy ship to USA
medicine in mexico pharmacies
Indian Pharmacy Abp: Best Indian pharmacy – Indian Pharmacy Abp
http://mexicanpharmacyacp.com/# buying prescription drugs in mexico online
reputable mexican pharmacies online
Online medicine home delivery: India pharmacy ship to USA – Indian pharmacy online
prescription drugs canada buy online: canadian pharmacy online – canada drug pharmacy
They understand the intricacies of international drug regulations.
can i buy lisinopril pill
The children’s section is well-stocked with quality products.
canadian online drugstore: canadian online pharmacy – pet meds without vet prescription canada
Very informative post.Really looking forward to read more. Awesome.
mexican rx online: mexican rx online – mexican border pharmacies shipping to usa
I cannot thank you enough for the article post.Much thanks again. Keep writing.
Thanks for sharing, this is a fantastic blog post.Really thank you! Awesome.
Indian pharmacy international shipping: indianpharmacy com – Indian pharmacy online
A round of applause for your blog article.Much thanks again.
mexican pharmacy acp mexican pharmacy acp mexican pharmacy acp
A pharmacy that keeps up with the times.
order cytotec without prescription
They are always proactive about refills and reminders.
Обнаружьте новые стратегии РЅР° автомате Ballon.: balloon казино – balloon game
balloon казино официальный сайт balloon казино Ргровые автоматы Ballon Р¶РґСѓС‚ СЃРІРѕРёС… героев.
Казино — РјРёСЂ азартных приключений.: balloon казино – balloon игра на деньги
Казино предлагает множество игровых автоматов.: balloon игра на деньги – balloon казино демо
I could not resist commenting. Very well written!Stop by my blog … tent heaters
We’re a group of volunteers and starting a new scheme in our community. Your site provided us with valuable information to work on. You have done an impressive job and our entire community will be thankful to you.
Крути барабаны Рё Р¶РґРё победы!: balloon game – balloon казино играть
whoah this blog is excellent i love studying your articles. Stay up the great paintings! You recognize, many persons are hunting around for this information, you can aid them greatly.
Major thanks for the blog.Really looking forward to read more. Fantastic.
I like the helpful information you provide in your articles. I will bookmark your blog and check again here frequently. I’m quite certain I?ll learn lots of new stuff right here! Best of luck for the next!
Really informative post.Thanks Again. Great.
Ballon — автомат СЃ захватывающим сюжетом.: balloon казино официальный сайт – balloon игра на деньги
cannabidiol oil cannabis tincture cbd for sleep problems dr oz cbd oil
Погрузитесь РІ РјРёСЂ азартных РёРіСЂ.: balloon казино демо – balloon game
A big thank you for your article post. Keep writing.
I’m extremely impressed with your writing skills andalso with the layout on your blog. Is this a paid theme or didyou customize it yourself? Either way keep up the nice quality writing, it is rareto see a great blog like this one these days.
Ballon — РёРіСЂР°, полная СЃСЋСЂРїСЂРёР·РѕРІ.: balloon игра на деньги – balloon казино официальный сайт
Fine forum posts. Appreciate it!essay about the help thesis statement maker professional business letter writing services
Thanks again for the post.Really looking forward to read more. Really Great.
Leading the charge in international pharmacy standards.
cheapest lisinopril 10 mg
A powerhouse in international pharmacy.
Удача всегда СЂСЏРґРѕРј, РєРѕРіРґР° играешь.: balloon игра – balloon игра на деньги
The drive-thru option is a lifesaver.
gabapentin and concerta interactions
Their worldwide reach ensures I never run out of my medications.
Рграйте РІ казино, наслаждайтесь каждым моментом.: balloon game – balloon игра на деньги
When I originally commented I clicked the „Notify me when new comments are added” checkboxand now each time a comment is added I get threee-mails with the same comment. Is there any way you can removepeople from that service? Thanks a lot!
I appreciate you sharing this blog.Much thanks again. Awesome.
Commonly Used Drugs Charts.
gabapentin capsules 300 mg
Always stocked with the best brands.
balloon игра balloon казино играть Сыграйте РЅР° деньги, почувствуйте азарт!
Please let me know where you got your theme. Bless you
k8vip: k8 th? dam – nha cai k8
dang nh?p alo789: dang nh?p alo789 – alo789
Very informative post. Will read on…
alo789hk alo 789 alo789hk
alo 789: alo789 chinh th?c – alo789 dang nh?p
https://88betviet.pro/# 88bet slot
Im grateful for the article post.Much thanks again. Really Great.
Thanks for every other wonderful post. The place else could anybody get that kind of information in such an ideal way of writing?I have a presentation subsequent week, and I’m at the search for such information.My blog post; fad diets
nha cai k8: link vao k8 – k8 th? dam
nha cai k8 k8 th? dam k8 th? dam
Mamibet merupakan situs judi slot pulsa terbaik yang memiliki beragam jenis permaiann judi slot online yang menguntungkan bagi semua pemain judi slot Indonesia.
https://k8viet.guru/# k8vip
alo 789: alo789in – alo 789 dang nh?p
Really appreciate you sharing this article.Thanks Again. Keep writing.
alo789hk: alo 789 – alo 789
k8vip link vao k8 link vao k8
Thanks again for the article.Thanks Again. Much obliged.
alo789 chinh th?c: alo 789 dang nh?p – alo 789
88bet slot 88bet slot keo nha cai 88bet
Good blog you have here.. It’s hard to find good quality writing like yours nowadays. I truly appreciate people like you! Take care!!
k8: k8 th? dam – k8vip
88bet 88bet 88bet
k8vip: nha cai k8 – k8 th? dam
88bet slot 188bet 88bet nha cai 88bet
canadian pharmacy ltd: canada pharmacy no prescription – online canadian pharmacy reviews
canadian valley pharmacy: certified canada pharmacy online – ordering drugs from canada
canadian pharmacy meds review: highest rated canadian online pharmacy – canadian pharmacy ed medications
https://indiamedfast.com/# india online pharmacy store
canadianpharmacyworld
reliable mexican pharmacies: mexican drug stores online – buying from online mexican pharmacy
canadian online pharmacy reviews Pharmacies in Canada that ship to the US canadian pharmacy online store
https://indiamedfast.com/# lowest prescription prices online india
https://mexicanpharminter.com/# mexican pharmacy online order
buying from canadian pharmacies
india online pharmacy store: IndiaMedFast.com – india online pharmacy store
adderall canadian pharmacy: most trusted canadian pharmacies online – canadian world pharmacy
https://mexicanpharminter.com/# mexican pharmacy online order
https://indiamedfast.com/# online medicine shopping in india
canadian pharmacy meds review
buying from online mexican pharmacy: MexicanPharmInter – Mexican Pharm International
https://mexicanpharminter.shop/# mexican pharmacy online
canadian pharmacies online
legitimate canadian pharmacies: highest rated canadian online pharmacy – canadian pharmacy 24 com
Im grateful for the blog article.Really thank you! Really Great.
https://interpharmonline.shop/# legitimate canadian pharmacy
india pharmacy without prescription buying prescription drugs from india cheapest online pharmacy india
https://mexicanpharminter.shop/# mexican pharmacy online
canadian pharmacy drugs online
https://indiamedfast.com/# India Med Fast
http://mexicanpharminter.com/# MexicanPharmInter
canadian pharmacies online
canadian online pharmacy: canadian drugstore online no prescription – canadian online drugstore
Mexican Pharm International Mexican Pharm International mexican pharmacy online
https://interpharmonline.com/# canada online pharmacy
canadian neighbor pharmacy
buying from online mexican pharmacy: reliable mexican pharmacies – mexican pharmacy online
canadian discount pharmacy: online canadian pharmacy no prescription – legit canadian online pharmacy
Generic100mgEasy: Viagra generic over the counter – Cheap Sildenafil 100mg
https://tadalafileasybuy.com/# TadalafilEasyBuy.com
kamagra 100mg kopen: kamagra kopen nederland – kamagra 100mg kopen
sildenafil online: Generic 100mg Easy – buy generic 100mg viagra online
https://generic100mgeasy.com/# Generic 100mg Easy
buy generic 100mg viagra online Generic Viagra online Generic 100mg Easy
Kamagra Kopen: kamagra gel kopen – kamagra jelly kopen
Generic 100mg Easy: buy generic 100mg viagra online – Generic100mgEasy
https://kamagrakopen.pro/# kamagra kopen nederland
https://kamagrakopen.pro/# kamagra gel kopen
An intriguing discussion is worth comment. I do think that you ought to write more on this issue, it may not be a taboo subject but usually people do not talk about such topics. To the next! Cheers!!
buy generic 100mg viagra online: sildenafil over the counter – Generic 100mg Easy
Thanks so much for the blog article.Much thanks again. Really Cool.
Kamagra Kopen Online: Officiele Kamagra van Nederland – Kamagra Kopen Online
I like reading through an article that can make men and women think. Also, thanks for allowing for me to comment!
Very good article post.Much thanks again. Fantastic.
Generic 100mg Easy: Generic 100mg Easy – best price for viagra 100mg
can you eat oranges with azithromycin – azithromycin for chlamydia over the counter zithromax interactions
http://generic100mgeasy.com/# Generic100mgEasy
Generic100mgEasy: buy Viagra over the counter – buy generic 100mg viagra online
Truly all kinds of wonderful information.writing literature essaysessays writer
Kamagra Kopen: Kamagra Kopen Online – Kamagra Kopen
KamagraKopen.pro: kamagra kopen nederland – kamagra pillen kopen
https://generic100mgeasy.shop/# buy generic 100mg viagra online
https://tadalafileasybuy.shop/# Tadalafil Easy Buy
https://tadalafileasybuy.shop/# Generic Cialis without a doctor prescription
Tadalafil Easy Buy: Tadalafil Tablet – cialis without a doctor prescription
You made some nice points there. I did a search on the subject matter and found most guys will approve with your blog.
KamagraKopen.pro: kamagra gel kopen – Kamagra
https://generic100mgeasy.shop/# best price for viagra 100mg
Generic100mgEasy: Generic100mgEasy – buy generic 100mg viagra online
https://kamagrakopen.pro/# kamagra gel kopen
https://kamagrakopen.pro/# kamagra gel kopen
TadalafilEasyBuy.com: Tadalafil Easy Buy – Cialis 20mg price
https://kamagrakopen.pro/# kamagra kopen nederland
TadalafilEasyBuy.com cialis without a doctor prescription TadalafilEasyBuy.com
пин ап: https://pinupkz.life/
пин ап зеркало – пин ап казино зеркало
Yoou really make it seem so easy along with your presentation however I find this matter
to be really one thing that I think I would by no means understand.
It seems too complex and extremely extensive for me.
I am having a look forward in your subsequent publish, I’ll try to get
the cling of it! https://menbehealth.wordpress.com/
пин ап зеркало: https://pinupkz.life/
Tadalafil price cialis without a doctor prescription cheapest cialis
пин ап казино официальный сайт: https://pinupkz.life/
пин ап – пин ап казино зеркало
kamagra 100mg kopen kamagra jelly kopen Kamagra Kopen Online
пин ап казино зеркало: https://pinupkz.life/
пин ап казино – пинап казино
пин ап казино официальный сайт – пин ап
pinup 2025 – пин ап казино официальный сайт
Generic 100mg Easy sildenafil over the counter Generic100mgEasy
пин ап казино официальный сайт: https://pinupkz.life/
пин ап казино – пин ап
пин ап казино официальный сайт – пин ап казино зеркало
пин ап: https://pinupkz.life/
https://kamagrapotenzmittel.shop/# Kamagra Oral Jelly
ApotheekMax: de online drogist kortingscode – Apotheek Max
Very well written information. It will be supportive to everyone who usess it, including me. Keep up the good work – looking forward to more posts.
http://apotekonlinerecept.com/# apotek online recept
https://apotheekmax.com/# online apotheek
Kamagra Gel Kamagra kaufen ohne Rezept Kamagra Oral Jelly kaufen
Fantastic blog article.Really looking forward to read more. Want more.
http://apotekonlinerecept.com/# Apotek hemleverans idag
Kamagra Original: kamagra – Kamagra Oral Jelly
http://apotekonlinerecept.com/# apotek online
Major thanks for the article.Really thank you! Great.
apotek pa nett: apotek pa nett – apotek online recept
https://apotheekmax.com/# ApotheekMax
http://apotekonlinerecept.com/# Apotek hemleverans recept
https://apotheekmax.com/# Online apotheek Nederland zonder recept
apotek pa nett Apoteket online Apotek hemleverans idag
Apotek hemleverans recept: apotek online – apotek pa nett
Great article post.Thanks Again. Much obliged.
online apotheek: Beste online drogist – Online apotheek Nederland zonder recept
https://apotekonlinerecept.com/# apotek online
https://apotheekmax.shop/# Online apotheek Nederland zonder recept
Apotek hemleverans idag: Apotek hemleverans recept – Apotek hemleverans recept
https://apotheekmax.shop/# Apotheek Max
online apotheek: Betrouwbare online apotheek zonder recept – Apotheek Max
http://apotekonlinerecept.com/# Apotek hemleverans idag
https://kamagrapotenzmittel.shop/# Kamagra Oral Jelly
Online apotheek Nederland met recept: Betrouwbare online apotheek zonder recept – online apotheek
Thanks for the blog post.Thanks Again. Really Great.
Apotek hemleverans idag: Apotek hemleverans idag – apotek online recept
Thanks so much for the blog post. Really Great.
ApotheekMax Online apotheek Nederland met recept de online drogist kortingscode
No matter if some one searches for his required thing, so he/she desires to be available that in detail, therefore that thing is maintained over here.
Kamagra Oral Jelly: Kamagra Oral Jelly kaufen – Kamagra Original
https://apotheekmax.com/# Apotheek Max
Kamagra Oral Jelly kaufen: kamagra – kamagra
Kamagra kaufen ohne Rezept Kamagra Gel Kamagra Oral Jelly kaufen
Apotheek online bestellen: Online apotheek Nederland zonder recept – de online drogist kortingscode
https://apotekonlinerecept.shop/# Apotek hemleverans idag
http://apotekonlinerecept.com/# Apotek hemleverans recept
https://apotheekmax.shop/# Apotheek online bestellen
Kamagra Oral Jelly: Kamagra Oral Jelly kaufen – Kamagra Oral Jelly
Kamagra online bestellen Kamagra Gel Kamagra Gel
mail order pharmacy india: india pharmacy – www india pharm
п»їbest mexican online pharmacies: pharmacies in mexico that ship to usa – Agb Mexico Pharm
https://gocanadapharm.com/# 77 canadian pharmacy
best rated canadian pharmacy: go canada pharm – canadian pharmacy ltd
Agb Mexico Pharm Agb Mexico Pharm п»їbest mexican online pharmacies
canada discount pharmacy: precription drugs from canada – online canadian drugstore
www india pharm: indian pharmacy paypal – www india pharm
https://agbmexicopharm.com/# mexico drug stores pharmacies
canadian drug prices: go canada pharm – canada drug pharmacy
pharmacies in mexico that ship to usa: Agb Mexico Pharm – Agb Mexico Pharm
best canadian pharmacy: go canada pharm – medication canadian pharmacy
https://wwwindiapharm.shop/# online pharmacy india
canadian pharmacy sarasota: go canada pharm – canada pharmacy 24h
canada drugstore pharmacy rx: GoCanadaPharm – online pharmacy canada
www india pharm: www india pharm – buy prescription drugs from india
www india pharm: pharmacy website india – www india pharm
https://wwwindiapharm.com/# www india pharm
buy canadian drugs: go canada pharm – canadian pharmacy phone number
www india pharm: www india pharm – best online pharmacy india
cheap canadian pharmacy online: GoCanadaPharm – canadian 24 hour pharmacy
mexican online pharmacies prescription drugs: Agb Mexico Pharm – Agb Mexico Pharm
canadian pharmacy uk delivery: cross border pharmacy canada – cheap canadian pharmacy online
canadian pharmacy 1 internet online drugstore: GoCanadaPharm – canadian neighbor pharmacy
canadian pharmacy meds: safe reliable canadian pharmacy – reputable canadian online pharmacies
https://wwwindiapharm.shop/# www india pharm
indian pharmacy online Online medicine home delivery indian pharmacy online
cheap canadian pharmacy online: onlinepharmaciescanada com – reputable canadian online pharmacies
mexican pharmaceuticals online: Agb Mexico Pharm – purple pharmacy mexico price list
https://wwwindiapharm.com/# www india pharm
www india pharm: п»їlegitimate online pharmacies india – online pharmacy india
Agb Mexico Pharm: Agb Mexico Pharm – Agb Mexico Pharm
canada pharmacy 24h go canada pharm ordering drugs from canada
http://agbmexicopharm.com/# Agb Mexico Pharm
canadian pharmacy uk delivery: reliable canadian pharmacy – safe canadian pharmacy
canadian valley pharmacy: GoCanadaPharm – buying from canadian pharmacies
Online medicine order: india online pharmacy – best online pharmacy india
Clom Fast Pharm: cost of generic clomid price – Clom Fast Pharm
Lisin Express: Lisin Express – Lisin Express
prednisone buy no prescription: prednisone 1mg purchase – mail order prednisone
AmOnlinePharm: AmOnlinePharm – generic amoxicillin over the counter
AmOnlinePharm: AmOnlinePharm – AmOnlinePharm
Clom Fast Pharm can i buy clomid cost of cheap clomid pills
https://clomfastpharm.shop/# can you get generic clomid pills
prednisone over the counter south africa: prednisone drug costs – Pred Pharm Net
Pred Pharm Net: cost of prednisone in canada – Pred Pharm Net
AmOnlinePharm: cost of amoxicillin 875 mg – amoxicillin 500mg cost
https://clomfastpharm.com/# order generic clomid pills
ZithPharmOnline: zithromax antibiotic without prescription – purchase zithromax z-pak
zithromax online pharmacy canada purchase zithromax online generic zithromax 500mg india
how to buy cheap clomid: can i buy generic clomid without insurance – clomid tablets
https://zithpharmonline.com/# zithromax tablets
Pred Pharm Net: prednisone 5mg daily – Pred Pharm Net
Lisin Express: lisinopril cost – Lisin Express
zithromax 500 price: zithromax for sale usa – zithromax for sale usa
Pred Pharm Net: Pred Pharm Net – Pred Pharm Net
lisinopril 80 mg daily: Lisin Express – Lisin Express
https://lisinexpress.shop/# lisinopril 5mg buy
Lisin Express Lisin Express cost of lisinopril in canada
I loved your blog post.Thanks Again. Much obliged.
Lisin Express: Lisin Express – Lisin Express
Lisin Express: Lisin Express – prescription drug lisinopril
online order prednisone 10mg: Pred Pharm Net – Pred Pharm Net
https://amonlinepharm.shop/# amoxicillin 500mg capsule
Lisin Express п»їbuy lisinopril 10 mg uk Lisin Express
Great, thanks for sharing this post.
medicine lisinopril 10 mg: Lisin Express – best lisinopril brand
lisinopril 20 mg best price: price of lisinopril 20 mg – Lisin Express
buy prednisone nz: Pred Pharm Net – purchase prednisone no prescription
https://amonlinepharm.com/# AmOnlinePharm
Muchos Gracias for your post. Really Cool.
Lisin Express order lisinopril online from canada Lisin Express
cheap clomid price: can i purchase generic clomid without prescription – how to get generic clomid without prescription
I loved your article.Really looking forward to read more. Really Cool.
https://amonlinepharm.shop/# AmOnlinePharm
AmOnlinePharm: can you buy amoxicillin uk – AmOnlinePharm
Clom Fast Pharm: can you get clomid tablets – can you buy generic clomid without prescription
http://amonlinepharm.com/# AmOnlinePharm
siteler bahis: casibom giris adresi – en bГјyГјk bahis siteleri casibom1st.com
sweet bonanza giris: sweet bonanza – sweet bonanza giris sweetbonanza1st.shop
Aw, this was a very good post. Taking the time and actual effort to produce a top notch article… but whatcan I say… I hesitate a whole lot and don’t manage to get nearly anything done.
en iyi deneme bonusu veren siteler casibom gercek para kazandД±ran casino oyunlarД± casibom1st.shop
sweet bonanza giris: sweet bonanza siteleri – sweet bonanza demo sweetbonanza1st.shop
en iyi yabancД± bahis siteleri: casibom 1st – yasal oyun siteleri casibom1st.com
sweet bonanza 1st: sweet bonanza siteleri – sweet bonanza demo sweetbonanza1st.shop
slot casino siteleri: casimo – slot casino siteleri casinositeleri1st.com
casino siteleri: casino siteleri – slot casino siteleri casinositeleri1st.com
2025 deneme bonusu veren bahis siteleri casibom giris bet turkiye casibom1st.shop
guvenilir casino siteleri: slot casino siteleri – casino siteleri casinositeleri1st.com
guvenilir casino siteleri: guvenilir casino siteleri – en iyi yabancД± bahis siteleri casinositeleri1st.com
slot casino siteleri: lisansl? casino siteleri – casino siteleri casinositeleri1st.com
https://casinositeleri1st.com/# lisansl? casino siteleri
yatД±rД±m bonusu veren siteler: casibom 1st – online kumar siteleri casibom1st.com
sweet bonanza siteleri: sweet bonanza oyna – sweet bonanza sweetbonanza1st.shop
sweet bonanza siteleri sweet bonanza oyna sweet bonanza giris sweetbonanza1st.com
sweet bonanza siteleri: sweet bonanza demo – sweet bonanza giris sweetbonanza1st.shop
lisansl? casino siteleri: casino siteleri – casino siteleri casinositeleri1st.com
Thanks so much for the article post. Cool.
guvenilir casino siteleri: slot casino siteleri – lisansl? casino siteleri casinositeleri1st.com
casino bet giriЕџ casibom guncel adres saДџlam bahis siteleri 2025 casibom1st.shop
bedbo: casibom giris adresi – casino bahis siteleri casibom1st.com
sweet bonanza demo: sweet bonanza siteleri – sweet bonanza giris sweetbonanza1st.shop
deneme bonusu veren siteler: casino siteleri 2025 – lisansl? casino siteleri casinositeleri1st.com
sweet bonanza yorumlar: sweet bonanza siteleri – sweet bonanza siteleri sweetbonanza1st.shop
gerГ§ek paralД± casino oyunlarД±: casinoda en Г§ok kazandД±ran oyun – lisansl? casino siteleri casinositeleri1st.com
Great blog. Really Cool.
https://casibom1st.shop/# casino giriЕџ
sweet bonanza demo: sweet bonanza giris – sweet bonanza 1st sweetbonanza1st.shop
Wow, great blog post.Really thank you! Great.
en iyi iddaa sitesi: casibom – yeni siteler bahis casibom1st.com
deneme bonusu veren siteler: guvenilir casino siteleri – deneme bonusu veren siteler casinositeleri1st.com
Thanks so much for the post.Thanks Again.
slot casino siteleri slot casino siteleri guvenilir casino siteleri casinositeleri1st.shop
sweet bonanza giris: sweet bonanza oyna – sweet bonanza giris sweetbonanza1st.shop
I think this is a real great post.Really thank you! Really Great.
http://casinositeleri1st.com/# deneme bonusu veren siteler
bilinmeyen siteler: casibom giris adresi – en iyi bahis uygulamasД± casibom1st.com
Us Mex Pharm: Mexican pharmacy ship to USA – mexican pharmacy
mexican pharmacy: usa mexico pharmacy – UsMex Pharm
http://usmexpharm.com/# Mexican pharmacy ship to USA
mexican pharmacy purple pharmacy mexico price list USMexPharm
usa mexico pharmacy: USMexPharm – certified Mexican pharmacy
certified Mexican pharmacy: mexico drug stores pharmacies – UsMex Pharm
certified Mexican pharmacy: Mexican pharmacy ship to USA – usa mexico pharmacy
https://usmexpharm.com/# Us Mex Pharm
UsMex Pharm: medicine in mexico pharmacies – USMexPharm
buying prescription drugs in mexico online: certified Mexican pharmacy – usa mexico pharmacy
purple pharmacy mexico price list: Mexican pharmacy ship to USA – Us Mex Pharm
https://usmexpharm.com/# UsMex Pharm
usa mexico pharmacy: Mexican pharmacy ship to USA – certified Mexican pharmacy
certified Mexican pharmacy: UsMex Pharm – Mexican pharmacy ship to USA
https://usmexpharm.shop/# mexican pharmacy
UsMex Pharm Mexican pharmacy ship to USA USMexPharm
certified Mexican pharmacy: mexican online pharmacies prescription drugs – mexican pharmacy
USMexPharm: Us Mex Pharm – Mexican pharmacy ship to USA
usa mexico pharmacy: UsMex Pharm – usa mexico pharmacy
UsMex Pharm: mexican pharmacy – USMexPharm
https://usmexpharm.shop/# certified Mexican pharmacy
usa mexico pharmacy: Mexican pharmacy ship to USA – mexican pharmacy
certified Mexican pharmacy: usa mexico pharmacy – certified Mexican pharmacy
http://usmexpharm.com/# Us Mex Pharm
mexican pharmacy: usa mexico pharmacy – buying prescription drugs in mexico
certified Mexican pharmacy usa mexico pharmacy USMexPharm
best online pharmacy india: mail order pharmacy india – best online pharmacy india
Very good article post.Really thank you! Awesome.
indianpharmacy com: USA India Pharm – indian pharmacies safe
top 10 online pharmacy in india: UsaIndiaPharm – india pharmacy mail order
Im thankful for the blog. Want more.
Online medicine home delivery USA India Pharm indian pharmacy online
USA India Pharm: mail order pharmacy india – USA India Pharm
USA India Pharm: online shopping pharmacy india – reputable indian pharmacies
Im obliged for the article. Great.
buy prescription drugs from india: mail order pharmacy india – UsaIndiaPharm
indian pharmacy: USA India Pharm – best india pharmacy
https://usaindiapharm.shop/# USA India Pharm
I loved your blog.Really looking forward to read more. Really Great.
UsaIndiaPharm: USA India Pharm – USA India Pharm
USA India Pharm UsaIndiaPharm USA India Pharm
best india pharmacy: top 10 online pharmacy in india – USA India Pharm
india pharmacy: indianpharmacy com – USA India Pharm
I appreciate you sharing this article.Really looking forward to read more. Really Great.
UsaIndiaPharm: online shopping pharmacy india – USA India Pharm
http://usaindiapharm.com/# UsaIndiaPharm
pharmacy website india UsaIndiaPharm USA India Pharm
best online pharmacy india: mail order pharmacy india – top 10 pharmacies in india
Im thankful for the post.Thanks Again. Great.
https://usaindiapharm.shop/# UsaIndiaPharm
Online medicine order: mail order pharmacy india – UsaIndiaPharm
USA India Pharm: india pharmacy – UsaIndiaPharm
USA India Pharm: best online pharmacy india – cheapest online pharmacy india
https://usaindiapharm.com/# buy medicines online in india
india online pharmacy USA India Pharm Online medicine home delivery
cheapest online pharmacy india: online pharmacy india – india pharmacy
online shopping pharmacy india: USA India Pharm – USA India Pharm
USA India Pharm: USA India Pharm – UsaIndiaPharm
http://usaindiapharm.com/# reputable indian online pharmacy
USA India Pharm: india pharmacy – indianpharmacy com
best india pharmacy: UsaIndiaPharm – п»їlegitimate online pharmacies india
indianpharmacy com: USA India Pharm – mail order pharmacy india
Hi there, just became alert to your blog through Google, and found that it is truly informative. I am gonna watch out for brussels. I’ll appreciate if you continue this in future. Numerous people will be benefited from your writing. Cheers!
Really enjoyed this article post. Really Great.
http://usaindiapharm.com/# USA India Pharm
USA India Pharm: UsaIndiaPharm – indian pharmacy online
UsaIndiaPharm: USA India Pharm – USA India Pharm
Looking forward to reading more. Great blog. Awesome.
buy medicines online in india: indian pharmacies safe – UsaIndiaPharm
https://usaindiapharm.shop/# USA India Pharm
indian pharmacy: Online medicine order – UsaIndiaPharm
Very informative blog post.Really thank you! Keep writing.
indian pharmacy paypal: Online medicine home delivery – UsaIndiaPharm
UsaIndiaPharm: USA India Pharm – indianpharmacy com
http://usaindiapharm.com/# UsaIndiaPharm
USA India Pharm: USA India Pharm – reputable indian online pharmacy
I think this is a real great article.Much thanks again. Cool.
online shopping pharmacy india: UsaIndiaPharm – india pharmacy mail order
Your manner of addressin this subject is both remarkable and also motivating.
https://usacanadapharm.shop/# my canadian pharmacy
Thanks for sharing, this is a fantastic post.Really looking forward to read more. Much obliged.
canada drugs online reviews: usa canada pharm – usa canada pharm
Really enjoyed this article.Much thanks again. Really Cool.
http://usacanadapharm.com/# usa canada pharm
USACanadaPharm: canadian pharmacy online ship to usa – canadian pharmacy
usa canada pharm my canadian pharmacy USACanadaPharm
canadian pharmacy online store: canada rx pharmacy world – USACanadaPharm
USACanadaPharm: canadian pharmacy 365 – 77 canadian pharmacy
https://usacanadapharm.shop/# canadian world pharmacy
This is one awesome blog.Much thanks again. Much obliged.
canadian pharmacy near me: canadian pharmacy – USACanadaPharm
usa canada pharm: USACanadaPharm – canadadrugpharmacy com
my canadian pharmacy rx usa canada pharm canadian pharmacy meds review
https://usacanadapharm.shop/# legitimate canadian pharmacy
USACanadaPharm: canada pharmacy online – canada drugs online reviews
http://usacanadapharm.com/# USACanadaPharm
I loved your article.Really thank you! Awesome.
best mail order pharmacy canada: safe canadian pharmacies – usa canada pharm
USACanadaPharm: online canadian pharmacy – USACanadaPharm
best canadian pharmacy to order from pharmacy wholesalers canada USACanadaPharm
Great, thanks for sharing this blog post.Much thanks again. Really Great.
canadian online drugs: usa canada pharm – usa canada pharm
canada drugs reviews: canadian family pharmacy – canadian neighbor pharmacy
http://usacanadapharm.com/# USACanadaPharm
USACanadaPharm canadian neighbor pharmacy reputable canadian online pharmacy
canadian pharmacy review: usa canada pharm – USACanadaPharm
northwest canadian pharmacy: certified canadian pharmacy – usa canada pharm
best canadian online pharmacy: legitimate canadian pharmacy – canadian pharmacies compare
canada drugs online medication canadian pharmacy canada discount pharmacy
http://usacanadapharm.com/# canada drugstore pharmacy rx
usa canada pharm: USACanadaPharm – USACanadaPharm
https://usacanadapharm.shop/# reddit canadian pharmacy
usa canada pharm: canadian pharmacy 24h com safe – canadian pharmacy com
usa canada pharm usa canada pharm canadianpharmacyworld
canadapharmacyonline: USACanadaPharm – usa canada pharm
https://olympecasino.pro/# olympe casino avis
olympe casino avis olympe casino en ligne
olympe casino avis olympe casino en ligne
Normally I do not learn post on blogs, but I wish to say that this write-up very compelled me to check out and do it! Your writing style has been amazed me. Thanks, quite great post.
olympe casino cresus: olympe casino cresus – olympe casino en ligne
clinton place apartments rentberry scam ico 30m$ raised apartments in frisco tx
casino olympe: olympe – olympe casino cresus
olympe casino en ligne: casino olympe – olympe casino cresus
Thanks a lot for the article.Really thank you! Awesome.
olympe casino cresus: olympe casino avis – olympe
https://olympecasino.pro/# casino olympe
olympe casino avis: casino olympe – olympe
casino olympe: olympe casino avis – olympe casino cresus
olympe casino avis: olympe casino avis – olympe casino avis
https://olympecasino.pro/# olympe casino
olympe casino cresus: olympe – olympe
casino olympe: olympe casino en ligne – casino olympe
olympe casino avis olympe casino avis
olympe casino avis: olympe casino – olympe casino avis
olympe casino en ligne: casino olympe – olympe
Aw, this was an exceptionally good post. Spending some time and actual effort to produce a good articleÖ but what can I sayÖ I hesitate a whole lot and don’t seem to get nearly anything done.
olympe casino en ligne: casino olympe – olympe casino en ligne
olympe casino: olympe casino cresus – olympe casino cresus
olympe casino cresus olympe casino cresus
so much superb information on here, : D.
olympe casino avis: olympe – olympe casino
olympe casino casino olympe
olympe casino avis olympe casino cresus
http://pharmafst.com/# Achat mГ©dicament en ligne fiable
pharmacie en ligne pas cher Pharmacie en ligne France acheter mГ©dicament en ligne sans ordonnance pharmafst.shop
Tadalafil sans ordonnance en ligne: cialis prix – Cialis sans ordonnance 24h tadalmed.shop
pharmacie en ligne france livraison internationale: Medicaments en ligne livres en 24h – pharmacie en ligne france livraison internationale pharmafst.com
hydrochlorothiazide danger hydrochlorothiazide tablets chlorthalidone vs hydrochlorothiazide
https://kamagraprix.com/# achat kamagra
Achetez vos kamagra medicaments: kamagra pas cher – kamagra gel
kamagra livraison 24h Kamagra pharmacie en ligne Kamagra pharmacie en ligne
pharmacie en ligne france livraison belgique: Pharmacies en ligne certifiees – pharmacie en ligne pas cher pharmafst.com
Cialis en ligne Acheter Cialis Tadalafil sans ordonnance en ligne tadalmed.com
pharmacie en ligne: Medicaments en ligne livres en 24h – pharmacie en ligne livraison europe pharmafst.com
Pharmacie Internationale en ligne: Pharmacie en ligne France – pharmacie en ligne livraison europe pharmafst.com
pharmacie en ligne Pharmacie en ligne France pharmacie en ligne avec ordonnance pharmafst.shop
https://kamagraprix.shop/# kamagra en ligne
kamagra 100mg prix: Kamagra Commander maintenant – kamagra oral jelly
acheter mГ©dicament en ligne sans ordonnance pharmacie en ligne france pas cher pharmacie en ligne sans ordonnance pharmafst.shop
acheter kamagra site fiable: kamagra 100mg prix – Achetez vos kamagra medicaments
achat kamagra: kamagra gel – Kamagra Commander maintenant
Kamagra pharmacie en ligne kamagra livraison 24h kamagra 100mg prix
https://tadalmed.shop/# cialis generique
cialis prix: Pharmacie en ligne Cialis sans ordonnance – Pharmacie en ligne Cialis sans ordonnance tadalmed.shop
acheter kamagra site fiable kamagra gel kamagra gel
Kamagra pharmacie en ligne: kamagra gel – kamagra en ligne
cialis prix: cialis generique – Cialis sans ordonnance 24h tadalmed.shop
Kamagra Commander maintenant: Kamagra Oral Jelly pas cher – kamagra 100mg prix
pharmacie en ligne sans ordonnance: Pharmacies en ligne certifiees – pharmacie en ligne avec ordonnance pharmafst.com
Acheter Cialis Acheter Viagra Cialis sans ordonnance Pharmacie en ligne Cialis sans ordonnance tadalmed.com
https://pharmafst.shop/# pharmacie en ligne france livraison belgique
Tadalafil sans ordonnance en ligne: Acheter Cialis – Tadalafil 20 mg prix sans ordonnance tadalmed.shop
http://kamagraprix.com/# Kamagra Commander maintenant
pharmacie en ligne fiable Medicaments en ligne livres en 24h Pharmacie en ligne livraison Europe pharmafst.shop
acheter kamagra site fiable: achat kamagra – Kamagra Oral Jelly pas cher
Kamagra Commander maintenant: Kamagra Commander maintenant – Kamagra Commander maintenant
https://kamagraprix.com/# Achetez vos kamagra medicaments
achat kamagra: Acheter Kamagra site fiable – kamagra 100mg prix
Pharmacie sans ordonnance: pharmacie en ligne pas cher – pharmacie en ligne france livraison belgique pharmafst.com
https://tadalmed.com/# Tadalafil 20 mg prix en pharmacie
Acheter Viagra Cialis sans ordonnance Cialis en ligne Cialis en ligne tadalmed.com
kamagra 100mg prix: Kamagra Commander maintenant – Acheter Kamagra site fiable
п»їpharmacie en ligne france: Livraison rapide – trouver un mГ©dicament en pharmacie pharmafst.com
kamagra 100mg prix kamagra gel Kamagra Oral Jelly pas cher
kamagra 100mg prix: achat kamagra – acheter kamagra site fiable
https://pharmafst.shop/# pharmacie en ligne pas cher
pharmacie en ligne livraison europe: pharmacie en ligne sans ordonnance – pharmacie en ligne france fiable pharmafst.com
Achat mГ©dicament en ligne fiable: Medicaments en ligne livres en 24h – п»їpharmacie en ligne france pharmafst.com
https://kamagraprix.com/# Kamagra pharmacie en ligne
Pharmacie en ligne livraison Europe: Medicaments en ligne livres en 24h – pharmacie en ligne livraison europe pharmafst.com
Профессиональный сервисный центр по ремонту бытовой техники с выездом на дом.
Мы предлагаем:ремонт крупногабаритной техники в москве
Наши мастера оперативно устранят неисправности вашего устройства в сервисе или с выездом на дом!
Tadalafil achat en ligne Tadalafil sans ordonnance en ligne Acheter Cialis 20 mg pas cher tadalmed.com
https://kamagraprix.shop/# kamagra pas cher
Awesome blog.Thanks Again. Fantastic.
https://tadalmed.shop/# Tadalafil 20 mg prix sans ordonnance
I haven?¦t checked in here for some time as I thought it was getting boring, but the last several posts are good quality so I guess I will add you back to my everyday bloglist. You deserve it my friend 🙂
http://kamagraprix.com/# Kamagra Commander maintenant
Im obliged for the article post.Much thanks again. Much obliged.
https://pharmafst.com/# Pharmacie sans ordonnance
pharmacie en ligne france livraison belgique: Meilleure pharmacie en ligne – pharmacie en ligne france livraison internationale pharmafst.com
pharmacie en ligne france pas cher: pharmacie en ligne pas cher – Pharmacie Internationale en ligne pharmafst.com
pharmacie en ligne livraison europe Pharmacies en ligne certifiees pharmacie en ligne france pas cher pharmafst.shop
https://tadalmed.shop/# Tadalafil 20 mg prix en pharmacie
I appreciate you sharing this post.Really thank you! Fantastic.
I loved your blog article.Thanks Again. Cool.
Muchos Gracias for your post.Really looking forward to read more. Cool.
RxExpressMexico: mexico pharmacies prescription drugs – RxExpressMexico
mexico drug stores pharmacies: mexican mail order pharmacies – mexican rx online
I appreciate you sharing this post.Much thanks again. Really Cool.
mexico pharmacy order online RxExpressMexico RxExpressMexico
https://rxexpressmexico.shop/# mexico pharmacies prescription drugs
reputable canadian pharmacy: Express Rx Canada – reliable canadian pharmacy reviews
I truly appreciate this article.Really looking forward to read more. Fantastic.
mexico pharmacies prescription drugs Rx Express Mexico mexico pharmacies prescription drugs
my canadian pharmacy: Express Rx Canada – canadian online pharmacy
indian pharmacy online: indian pharmacy online – Medicine From India
We are looking for experienced people that might be interested in from working their home on a full-time basis. If you want to earn $200 a day, and you don’t mind developing some short opinions up, this is the perfect opportunity for you!
medicine courier from India to USA: top online pharmacy india – indian pharmacy online shopping
MedicineFromIndia indian pharmacy indian pharmacy online
medicine courier from India to USA: MedicineFromIndia – Medicine From India
https://rxexpressmexico.com/# Rx Express Mexico
indian pharmacy online shopping: indian pharmacy online shopping – indian pharmacy online
indian pharmacy online Medicine From India Medicine From India
Rx Express Mexico: mexican rx online – mexican online pharmacy
пин ап казино официальный сайт пин ап казино официальный сайт пин ап казино официальный сайт
Very good blog.Much thanks again. Cool.
pin up az: pin up casino – pin up az
pin up azerbaycan: pin up az – pin up casino
https://vavadavhod.tech/# вавада зеркало
вавада официальный сайт vavada вход vavada вход
pin up: pin-up – pin-up casino giris
vavada casino: вавада казино – вавада казино
вавада: vavada – vavada casino
pinup az: pin-up – pin up az
pin up az pin-up pin up
Very informative article.Really looking forward to read more. Great.
пинап казино: пин ап вход – пин ап казино
вавада: вавада зеркало – вавада казино
vavada: vavada – вавада
вавада зеркало вавада вавада казино
pin up az: pin up – pin up
http://pinupaz.top/# pin-up
пин ап вход: pin up вход – пин ап казино
https://vavadavhod.tech/# vavada вход
vavada вавада официальный сайт вавада
https://pinuprus.pro/# пин ап зеркало
Thanks a lot for the article post.Really thank you!
пин ап вход: пинап казино – pin up вход
pin up pin up az pinup az
vavada casino: vavada – вавада официальный сайт
пин ап казино: пин ап казино – пинап казино
Great blog.Really looking forward to read more. Really Great.
https://pinuprus.pro/# пин ап зеркало
pin up вход пинап казино пин ап вход
pin up azerbaycan: pin up azerbaycan – pin up azerbaycan
pin up azerbaycan: pin-up casino giris – pin up azerbaycan
Hello there! Do you use Twitter? I’d like to follow you ifthat would be okay. I’m definitely enjoying yourblog and look forward to new updates.
https://pinupaz.top/# pin up az
vavada casino vavada casino вавада официальный сайт
I really enjoy the blog.Really looking forward to read more. Great.
pin up вход пин ап вход пин ап зеркало
pin up az: pin-up casino giris – pin up casino
vavada: вавада официальный сайт – vavada casino
vavada: вавада – vavada
http://pinuprus.pro/# пинап казино
wow, awesome article.Thanks Again.
pinup az [url=https://pinupaz.top/#]pin up azerbaycan[/url] pin up az
http://pinuprus.pro/# пин ап вход
вавада [url=https://vavadavhod.tech/#]вавада казино[/url] вавада официальный сайт
пин ап казино: пинап казино – пин ап вход
Really appreciate you sharing this article.Really looking forward to read more. Keep writing.
вавада официальный сайт: вавада зеркало – вавада казино
Enjoyed every bit of your post.Really thank you! Awesome.
pin up casino: pin up – pin up azerbaycan
пин ап вход: pin up вход – пин ап казино официальный сайт
vavada casino [url=http://vavadavhod.tech/#]вавада официальный сайт[/url] вавада казино
пин ап вход: пин ап вход – pin up вход
Thanks-a-mundo for the article. Want more.
pin-up casino giris: pin-up – pin up azerbaycan
пинап казино: пин ап зеркало – пин ап казино официальный сайт
vavada casino [url=http://vavadavhod.tech/#]vavada[/url] вавада зеркало
вавада официальный сайт: вавада зеркало – vavada
pin up azerbaycan: pin up azerbaycan – pin up az
pin up вход [url=http://pinuprus.pro/#]пин ап вход[/url] пин ап зеркало
пин ап вход: пин ап зеркало – пинап казино
http://pinuprus.pro/# пинап казино
pin-up [url=https://pinupaz.top/#]pin-up[/url] pin up azerbaycan
пин ап казино официальный сайт: pin up вход – пин ап казино
pin up casino: pin up – pin up casino
пин ап вход [url=https://pinuprus.pro/#]пин ап вход[/url] pin up вход
вавада казино: vavada вход – вавада официальный сайт
I wanted to thank you for this fantastic read!! I definitely enjoyed every little bit of it. I have you saved as a favorite to check out new things you postÖ
пин ап зеркало: pin up вход – пин ап казино официальный сайт
http://vavadavhod.tech/# vavada casino
пин ап вход: пин ап казино – пин ап казино официальный сайт
There’s certainly a lot to learn about this subject. I really like all the points you’ve made.
pinup az: pin up casino – pin up azerbaycan
http://pinupaz.top/# pin up azerbaycan
pin up: pin up casino – pin up
Hey There. I discovered your blog the use of msn. That is a really well written article.I will be sure to bookmark it and return to learn extra of your usefulinfo. Thank you for the post. I’ll definitely comeback.
vavada [url=https://vavadavhod.tech/#]vavada вход[/url] вавада
http://vavadavhod.tech/# vavada вход
vavada вход: vavada вход – vavada вход
вавада: вавада казино – вавада зеркало
I’m not sure exactly why but this blog is loading incredibly slow for me. Is anyone else having this issue or is it a problem on my end? I’ll check back later and see if the problem still exists.
vavada вход [url=http://vavadavhod.tech/#]вавада зеркало[/url] вавада казино
Heya i am for the first time here. I came across this board and I findIt truly helpful & it helped me out a lot. I’m hopingto present something again and help others such as you helpedme.
vavada casino: вавада казино – vavada вход
Incredible! This blog looks just like my old one!It’s on a completely different topic but it has pretty much the same layout and design. Superb choice of colors!
Great blog.Really looking forward to read more. Awesome.
Hi there, its good paragraph concerning media print, we all be familiar with media is a wonderful source ofinformation.
пин ап казино официальный сайт [url=http://pinuprus.pro/#]пин ап зеркало[/url] пин ап зеркало
http://pinuprus.pro/# пин ап зеркало
vavada: vavada вход – вавада
It’s going to be finish of mine day, however before finish I am reading this great article to increase my know-how.
Aw, this was a really nice post. Finding the time and actual effort to make a good article… but what can I say… I procrastinate a whole lot and never seem to get anything done.
I have not checked in here for a while because I thought it was getting boring, but the last few posts are great quality so I guess I will add you back to my daily bloglist. You deserve it my friend 🙂
asus zen pro book 15 2019 avis Fiche Technique Tracteur Renault 55 1969 Avac Cabine Gratuitement la rochelle travel guide book
pinup az pinup az pin-up
pin up casino: pin up casino – pinup az
vavada casino: vavada casino – вавада официальный сайт
Attractive section of content. I just stumbled upon your blog andin accession capital to assert that I get in fact enjoyed account your blog posts.Anyway I’ll be subscribing to your augment and even I achievement you access consistently fast.
https://pinupaz.top/# pin up az
пинап казино пин ап зеркало пин ап казино официальный сайт
pinup az: pin up az – pin up az
пин ап вход: пин ап зеркало – pin up вход
I’ll right away clutch your rss as I can’t find your e-mail subscription link or e-newsletter service.Do you have any? Kindly permit me understand so that I may just subscribe.Thanks.
https://pinuprus.pro/# пин ап казино официальный сайт
Heya i’m for the first time here. I foundthis board and I find It really useful & it helped me out a lot.I hope to give something back and help others like you helped me.
vavada вход вавада vavada casino
hire someone to write college essay essay writer org write academic essay
pin-up casino giris pinup az pin-up
I truly appreciate this article post.Really thank you! Keep writing.
http://vavadavhod.tech/# vavada
vavada casino: vavada – vavada вход
пин ап казино pin up вход пин ап казино официальный сайт
Hello, just wanted to say, I liked this post.It was inspiring. Keep on posting!
pin-up casino giris: pin up – pin up casino
http://vavadavhod.tech/# vavada
пин ап зеркало пин ап зеркало пинап казино
пин ап вход: пин ап казино официальный сайт – пин ап вход
pin-up casino giris: pin up – pin up az
I cannot thank you enough for the article post.Much thanks again. Will read on…
pin up az: pin up casino – pin up azerbaycan
пин ап казино официальный сайт pin up вход пин ап вход
вавада казино: вавада казино – vavada casino
http://vavadavhod.tech/# вавада официальный сайт
Fantastic blog article.Thanks Again. Awesome.
vavada casino: vavada – вавада
Looking forward to reading more. Great post.Really thank you! Cool.
https://vavadavhod.tech/# вавада зеркало
A big thank you for your article.Really looking forward to read more. Fantastic.
online Cialis pharmacy: affordable ED medication – secure checkout ED drugs
Viagra without prescription [url=http://maxviagramd.com/#]discreet shipping[/url] discreet shipping
When someone writes an piece of writing he/she keeps the thoughtof a user in his/her brain that how a user can be awareof it. Thus that’s why this article is perfect. Thanks!
wow, awesome blog.Much thanks again.
fast Viagra delivery: same-day Viagra shipping – secure checkout Viagra
Itís hard to find knowledgeable people in this particular topic, but you sound like you know what youíre talking about! Thanks